A whirlwind tour of DevTools tips and tricks
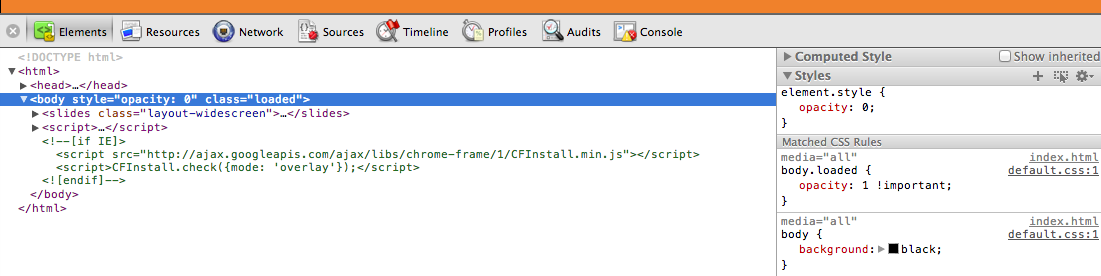
- Part I: DevTools tips, tricks and awesome features (video)
- Part II: Extending DevTools, Debug Protocol, Extensions, ... (video)
- Follow along @ bit.ly/devtools-tips
Resource loaders are great, except.. when they hide the resources excessively.
"You get used to it. I don't even see the waterfall. All I see is server stalls, queued requests, ..." :-)
If you're shuffling binary data, you'll need a custom viewer...
What's a HAR? HTTP Archive format!
# quick waterfall viewer! $ gem install har $ har trace.har # capture a HAR file from the CLI $ phantomjs examples/netsniff.js http://www.igvita.com/ > igvita.har # run YSlow on the waterfall $ npm install yslow -g $ yslow --info basic --format plain igvita.har size: 67.0K (67000 bytes)
60FPS? That's for games and stuff, right?
{"startTime": 1349100319000.9, "data": { "scriptName": "http://dnn506yrbagrg.cloudfront.net/pages/scripts/0011/6381.js?374750", "scriptLine": 1 }, "endTime": 1349100319001.1, "type": "FunctionCall", "usedHeapSize": 24341620, // ...
#protip: install PageSpeed Insights for up-to-date recommendations on performance best practices
var category = chrome.experimental.devtools.audits.addCategory("Readability", 2); category.onAuditStarted.addListener(function(results) { var details = results.createResult("Details..."); var styles = details.addChild("2 styles with small font"); var elements = details.addChild("3 elements with small font"); results.addResult("Font Size (5)", "5 elements use font size below 10pt", results.Severity.Severe, details); results.addResult("Contrast", "Text should stand out from background", results.Severity.Info); });
Example audit plugin. Are you doing repetitive tasks you can automate? Audit to the rescue!
chrome.devtools.panels.create("Font Picker", "FontPicker.png", "Panel.html", function(panel) { ... }); chrome.devtools.panels.elements.createSidebarPane("Font Properties", function(sidebar) { sidebar.setPage("Sidebar.html"); sidebar.setHeight("8ex"); });
Make DevTools your own, customize the color scheme.
Connect your Android device via USB to the desktop & view and debug the code executing on the device. Or, connect to a remote Chrome instance!
$> adb forward tcp:9222 localabstract:chrome_devtools_remote $> chrome.exe --remote-debugging-port=9222
All data is generated and received via a WebSocket!
resp = http_get_json('http://localhost:9292/json') ws = Faye::WebSocket::Client.new(resp.first['webSocketDebuggerUrl']) ws.onopen = lambda do |event| ws.send JSON.dump({id: 1, method: 'Network.enable'}) ws.send JSON.dump({ id: 2, method: 'Page.navigate', params: {url: 'http://twitter.com/#!/search/chrome?q=devtools&' + rand(100).to_s} }) end ws.onmessage = lambda do |event| p [:new_message, JSON.parse(event.data)] end
You can consume this data in real-time...
"method":"Network.responseReceived", "params":{ "type":"XHR", "response":{ "url":"http://api.twitter.com/1/trends/available.json?lang=en", "status":200, "statusText":"OK", "mimeType":"application/json", "connectionReused":false, "fromDiskCache":false, "timing":{ "requestTime":1333830921.9814498, "connectStart":1, "receiveHeadersEnd":234 // ...
PonyDebugger is a client library and gateway server combination that uses Chrome Developer Tools on your browser to debug your application's network traffic and managed object contexts.
There are so many things to take into account...
# Install extension @ https://chrome.google.com/webstore/detail/channimfdomahekjcahlbpccbgaopjll $ path/to/chrome --enable-benchmarking $ appify your-shell-script.sh "Your App Name" # OSX tip
Ex, Chrome learns subresource domains:
console.time("update"); update(); console.timeEnd("update"); console.time("render"); update(); console.timeEnd("render");
Slides @ bit.ly/devtools-tips